
This article will cover how to create and destroy a GameObject in Unity.
Setup
Before we start, we’ll need to set up 2 GameObjects, a CubeSpawner and a Capsule object, and a Cube Prefab in our Scene.
CubeSpawner
- Right-click the Hierarchy window > Create Empty > Rename it to “CubeSpawner” and set its position at the origin.
- Create and attach a script to the CubeSpawner. Call it “CubeSpawnerScript”.
Capsule
- Right-click the Hierarchy window > 3D Object > Capsule and move it about 4 units to the right so it displays on the right side of the Game View.
- Create and attach a script to the capsule. Call it “CapsuleScript”.
Cube Prefab
- Right click the Hierarchy window > 3D Object > Cube.
- Create a Prefab folder > drag and drop the Cube into the Prefab folder > delete the Cube in the Hierarchy window.

- Prefab: A Prefab is a shared instance of a GameObject. They are denoted with a blue cube icon. Prefabs are useful for creating duplicates of a GameObject that will be used across an entire project.
CubeSpawnerScript
With the setup complete, we’ll start the implementation of the CubeSpawnerScript.
Pseudocode
Let’s write some pseudocode to clarify what we want this script to do.
If the player taps the Spacebar
Create a Cube
Create the Cube
Now, we translate this into code:
- To get a player input, we use the Input class which has a GetKey() method to determine if a player tapped the correct key.
- To create the Cube, we can use the Instantiate() method. Instantiate() produces a clone of a designated GameObject at a specified position and rotation.
- First, we need a reference to the Cube we made earlier.
- Then, we need a position to place it. We’ll use the CubeSpawner’s position as the reference point.
- Lastly, we need a Quaternion. We’ll use the Quaternion.identity. Simply put, it means “no rotation” and is the Quaternion you’ll use the most often, but you can learn more about Quaternions at https://docs.unity3d.com/ScriptReference/Quaternion.html.
With the above explanation, try implementing the script on your own. When you’re done or if you get stuck, you can look at the code below:

This is the code that I’m using to create the Cube. Don’t forget to drag the CubePrefab into the CubeSpawnerScript before you run the script. Here’s the result in the Game View:

CapsuleScript
Pseudocode
Now, we can work on the CapsuleScript. Again, we write pseudocode to clarify what we want the script to do.
If the player taps backspace,
Destroy the Capsule
Destroy the Capsule
Translating the pseudocode:
- We use the Input class to check the player’s input.
- To destroy the Capsule, we use the Destroy() method. It takes a GameObject as an argument and removes it from the Scene.
This is pretty straightforward, so here’s the implementation:
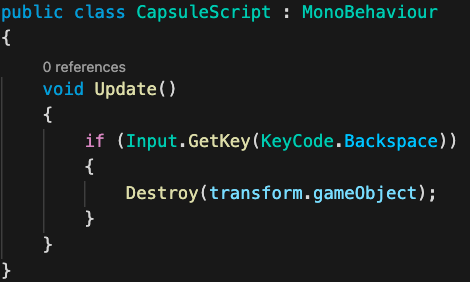
Now, when you tap the Backspace key, the capsule will disappear!

Congrats! You can now add and remove GameObjects in your game!