
In Unity, it’s nice to structure code such that GameObjects have their own procedures to access and modify their information. This idea is known as object-oriented programming. However, what if other entities need to interact and utilize that GameObject’s methods? This article will cover how Unity uses the GetComponent method to do this.
GetComponent<Type>()
GetComponent is a method of the GameObject class that takes a Type class, usually a component name, and returns that component. With a reference to the component, we can access and modify its properties through a script.
Here’s a visual guide for understanding how GetComponent operates. We’ll use an example of changing the Mass of a GameObject to 5:

- Select the desired GameObject.
- Look at the GameObject’s components in the Inspector.
- Remember the name of the component to access and the property to edit.
Script Code to Reassign Property Value
With the above information, we translate this thought process into code.
transform.gameObject.GetComponent<Rigidbody>().mass = 5f;
In code, using GetComponent will look like a daisy chain of reference calls until you get to the desired property that you want to modify.
Using GetComponent to Access a Script’s Methods

Now imagine that we have a game where we have a wall with limited durability. We want the wall to break after being hit 3 times.
We can construct a scene where we’ll have 3 sphere GameObjects drop onto the wall to break it. The wall GameObject will have a script that tracks its durability. The spheres will have a script to access the wall’s durability variable upon collision and reduce its durability.
WallScript Setup
First, we’ll set up a rectangular GameObject near the bottom of the scene.
In WallScript, we’ll need a variable to track the wall’s durability. Then we’ll need a public method for other GameObjects to access and reduce the wall’s durability. Finally, we’ll destroy the wall if its durability hits 0.
Code

SphereScript Setup
We’ll set up 3 spheres near the top of the scene above the wall. For movement, we’ll use the Rigidbody component’s UseGravity property to make our spheres fall downward.
In SphereScript, we’ll need to listen for an OnCollisionEnter call. Make sure the spheres and the wall all have Rigidbody/Collider components. Once the method is called, we’ll need to find out if the sphere hit the wall, and if it did, damage the wall using the wall’s public method Damage.
Actual Code

A good check to do when dealing with GetComponent is to ensure its return value is not null. Otherwise, problems can arise in your game.
Result
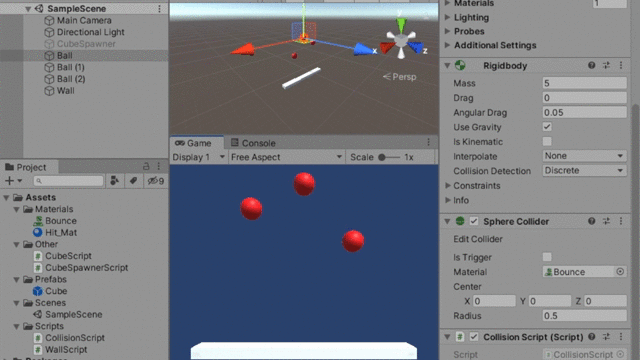
The wall is destroyed successfully! Hopefully, this knowledge helps you in your Unity journey! Good luck!